Arrays
An array is a container object that holds a fixed number of values of a single type. The length of an array is established when the array is created. After creation, its length is fixed. You have seen an example of arrays already, in the
main
method of the "Hello World!" application. This section discusses arrays in greater detail.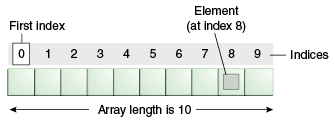
An array of 10 elements.
Each item in an array is called an element, and each element is accessed by its numerical index. As shown in the preceding illustration, numbering begins with 0. The 9th element, for example, would therefore be accessed at index 8.
The following program,
ArrayDemo
, creates an array of integers, puts some values in the array, and prints each value to standard output.class ArrayDemo { public static void main(String[] args) { // declares an array of integers int[] anArray; // allocates memory for 10 integers anArray = new int[10]; // initialize first element anArray[0] = 100; // initialize second element anArray[1] = 200; // and so forth anArray[2] = 300; anArray[3] = 400; anArray[4] = 500; anArray[5] = 600; anArray[6] = 700; anArray[7] = 800; anArray[8] = 900; anArray[9] = 1000; System.out.println("Element at index 0: " + anArray[0]); System.out.println("Element at index 1: " + anArray[1]); System.out.println("Element at index 2: " + anArray[2]); System.out.println("Element at index 3: " + anArray[3]); System.out.println("Element at index 4: " + anArray[4]); System.out.println("Element at index 5: " + anArray[5]); System.out.println("Element at index 6: " + anArray[6]); System.out.println("Element at index 7: " + anArray[7]); System.out.println("Element at index 8: " + anArray[8]); System.out.println("Element at index 9: " + anArray[9]); } }
The output from this program is:
Element at index 0: 100 Element at index 1: 200 Element at index 2: 300 Element at index 3: 400 Element at index 4: 500 Element at index 5: 600 Element at index 6: 700 Element at index 7: 800 Element at index 8: 900 Element at index 9: 1000
Advantage of Java Array
- Code Optimization: It makes the code optimized, we can retrieve or sort the data easily.
- Random access: We can get any data located at any index position.
Disadvantage of Java Array
- Size Limit: We can store only fixed size of elements in the array. It doesn't grow its size at runtime. To solve this problem, collection framework is used in java.
Types of Array in java
There are two types of array.
- Single Dimensional Array
- Multidimensional Array
Single Dimensional Array in java
Syntax to Declare an Array in java
- dataType[] arr; (or)
- dataType []arr; (or)
- dataType arr[];
Instantiation of an Array in java
- arrayRefVar=new datatype[size];
Example of single dimensional java array
Let's see the simple example of java array, where we are going to declare, instantiate, initialize and traverse an array.
class Testarray{
public static void main(String args[]){
int a[]=new int[5]; //declaration and instantiation
a[0]=10; //initialization
a[1]=20;
a[2]=70;
a[3]=40;
a[4]=50;
//printing array
for(int i=0;i<a.length;i++)//length is the property of array
System.out.println(a[i]);
}}
Output:
10 20 70 40 50
Multidimensional array in java
In such case, data is stored in row and column based index (also known as matrix form).Syntax to Declare Multidimensional Array in java
- dataType[][] arrayRefVar; (or)
- dataType [][]arrayRefVar; (or)
- dataType arrayRefVar[][]; (or)
- dataType []arrayRefVar[];
Example to instantiate Multidimensional Array in java
int[][] arr=new int[3][3];//3 row and 3 columnExample of Multidimensional java arrayLet's see the simple example to declare, instantiate, initialize and print the 2Dimensional array.class Testarray3{public static void main(String args[]){ //declaring and initializing 2D array int arr[][]={{1,2,3},{2,4,5},{4,4,5}}; //printing 2D array for(int i=0;i<3;i++){for(int j=0;j<3;j++){ System.out.print(arr[i][j]+" "); }System.out.println(); } }}Output:1 2 32 4 5 4 4 5
No comments:
Post a Comment